Introduction
Mixins provide a flexible and powerful way to create behaviors within classes. Mixins in Python allow developers to inject reusable codes into multiple classes. This promotes a modular, maintainable codebase. This blog will examine the concept of mixing, focusing on “In_a_dndmixin_drag”, and “Python Mixin” and “Maya Mixin.”
Understanding In_a_dndmixin_drag
A mixin class is a type of object that can provide methods to another class without being an independent object. Mixins are different from traditional inheritance where a class inherits properties and behaviors only from one parent. They allow the addition of functionalities that come from multiple sources. It encourages reuse of code and separation of concern, which makes the codebase easier to manage and scale.
The Concept of In_a_dndmixin_drag
The term “In_a_dndmixin_drag”, can be understood as a journey metaphorically through the complexity and elegance of Python mixins. As a Dungeons & Dragons dragon is a powerful creature with many abilities, a Python mixin can enhance classes’ capabilities without inheritance.
Python Mixins
Python is a dynamic and flexible language that makes it a great platform to implement mixins.
Let’s look at a practical example to see implimentation of mixins in Python.
The Maya Mixin
In the realm of 3D graphics and animation, Autodesk Maya is a leading software, and the concept of mixins can be applied to create modular and reusable components. Let’s consider an example where we create a `Maya Mixin` to handle common operations in Maya using Python’s `maya.cmds` module.
Examples
class FlyMixin:
def fly(self):
return "Flying high in the sky!"
class SwimMixin:
def swim(self):
return "Swimming deep in the ocean!"
class Dragon(FlyMixin, SwimMixin):
def roar(self):
return "Roaring with might!"
Creating an instance of Dragon
dragon = Dragon()
print(dragon.fly()) # Output: Flying high in the sky!
print(dragon.swim()) # Output: Swimming deep in the ocean!
print(dragon.roar()) # Output: Roaring with might!
In this example, the `Dragon` class inherits behaviors from both `FlyMixin` and `SwimMixin`,
demonstrating use of mixins to compose a class with multiple functionalities.
“`python
import maya.cmds as cmds
class MayaMixin:
def create_sphere(self, name, radius):
cmds.polySphere(name=name, radius=radius)
return f”Sphere ‘{name}’ with radius {radius} created.”
def create_cube(self, name, width):
cmds.polyCube(name=name, width=width)
return f”Cube ‘{name}’ with width {width} created.”
class MayaObject(MayaMixin):
def init(self, name):
self.name = name
Creating an instance of MayaObject
maya_obj = MayaObject(“myObject”)
print(maya_obj.create_sphere(“mySphere”, 5)) # Output: Sphere ‘mySphere’ with radius 5 created.
print(maya_obj.create_cube(“myCube”, 3)) # Output: Cube ‘myCube’ with width 3 created.
In this example, the `MayaMixin` class encapsulates methods to create basic 3D shapes in Maya. The `MayaObject` class can then utilize these methods, showcasing how mixins facilitate code reuse and abstraction in a specialized domain like 3D modeling.
html
- Mixins: Advantages and Applications
- Modularity: By isolating functionalities into mixins, the code gains modularity, making it easier for developers to manage. We can make Changes in the mixin class without impacting the rest of the codebase.
- Improved Flexibility: Mixins facilitate the creation of dynamic classes, resulting in highly customizable code architectures.
- Streamlined Inheritance: Mixins provide a straightforward approach to enhance the functionalities of classes
Game Mechanics and In-A-in_a_dndmixin_drag
Read in depth article on Game Mechanics and In-A-in_a_dndmixin_drag
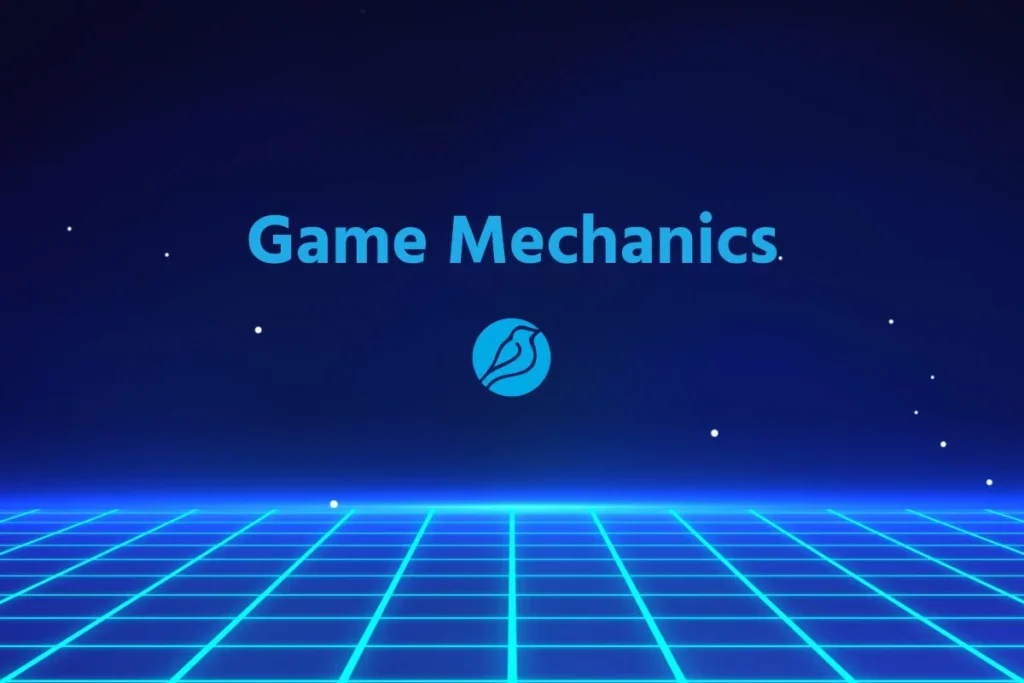
Game mechanics are the rules and procedures that guide playing the game of Dungeons & Dragons. They include rules on character generation, combat, using magic spells, and making skill checks. They put a framework around how players can engage with one another and the environment in a game so that play goes smoothly and fairly. For instance, spellcasting mechanics detail the preparation and execution of the spell and its effects. Combat mechanics determine whether or not an attack was successful and how much damage it inflicts by rolling dice. In this way, a good system of mechanics will ensure the integrity of the game so that it allows all players an equal opportunity to enjoy both narrative and action.
Difference between mixin and interface
A mixin and an interface are both programming constructs, Which are used to achieve modularity and reusability, but they serve different purposes and are implemented differently.
Mixin
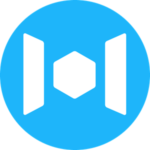
Definition: of mixin is as a class that provides certain additional methods to other classes and hence should not used independently. It adds certain new features to a class without using inheritance.
Structure: A structure of mixins is usually created by a class having some methods that can be mixed with other classes. In languages such as Python, this mechanism is achieved through multiple inheritances.
Usage: Mixins come in handy when we need to share some methods between more than one class. They should not be instantiated themselves. For example, a mixin may provide logging or serialization which can be added to any class.
Example
class LogMixin: def log(self, message): print(f’Log: {message}’) class MyClass(LogMixin): def do_something(self):
self.log(‘Doing something!’) obj = MyClass()
obj.do_something() # Output: Log: Doing something!
Interface
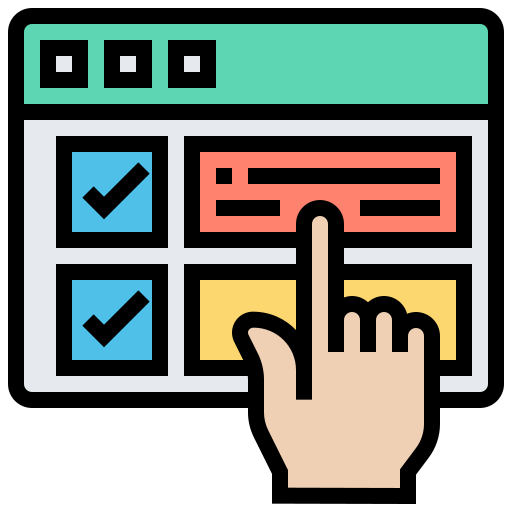
Definition: An interface in object-oriented programming is a construct where the class must implement a contract that describes methods to be implemented without providing implementations for the methods. One can consider an interface as a set of methods to be used in any class that implements that interface.
Implementation: In many languages, including Java and C#, interfaces are created using certain keywords that provide an interface definition. A class that implements an interface, therefore, must implement all methods defined by that interface.
Usage: The use of an interface is to define a contract for behavior to implement by more than one class, such that all of them implement the same set of method signatures. If used properly, this can be quite effective when it comes to polymorphism and decoupling your code.
Example
interface Animal { void makeSound(); } class Dog implements Animal { public void makeSound() { System.out.println(“Bark”); } } class Cat implements Animal { public void makeSound() { System.out.println(“Meow”); } } public class Main { public static void main(String[] args) { Animal dog = new Dog(); dog.makeSound(); // Output: Bark Animal cat = new Cat(); cat.makeSound(); // Output: Meow } }
Best Practices for Utilizing Mixins
- Single Responsibility Principle: Each mixin should have a distinct and well-defined purpose. Avoid creating extensive mixin classes that attempt to handle too many functions.
- Prevent Name Conflicts: When merging several mixins, be cautious with method names to prevent overlaps.
- roperly document mixin classes and methods to ensure their ease of use by other developers.
- Thoroughly test mixins to confirm they operate as intended.
Summary of In_a_dndmixin_drag
Mixins serve as a powerful asset for Python developers. They enable the creation of modular, reusable, and maintainable code. Whether you’re working with a fantastical setting involving dragons through “In_a_dndmixin_drag” or diving into the intricate world of 3D graphics with “Mayamixin,” mixins can bolster your code’s flexibility, promote reuse, and simplify inheritance hierarchies. This ultimately enhances the efficiency and enjoyment of your development journey.
Read More articles on Technology, or for more categories visit USA Up Trend.